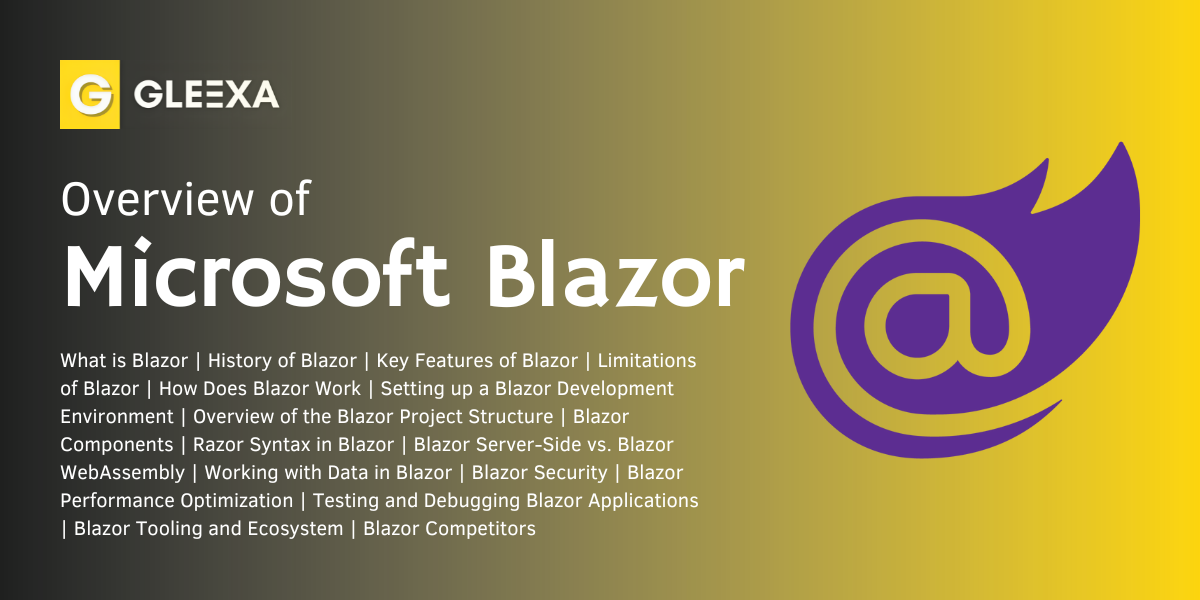
As a C# developer, the idea of using my existing skills to build interactive web apps instead of wrestling with JavaScript is like a dream come true. I can’t tell you how many times I’ve pulled my hair out debugging JS code. No offense to JavaScript, but C# just clicks better in my brain!
Here’s the deal with Blazor – it lets you build powerful web apps using C# and .NET…but runs that code directly in the browser! Wait, how does that sorcery happen, you ask? Well, my friend, it uses this nifty little thing called WebAssembly that essentially takes your .NET code and runs it client-side after it’s compiled into a format web browsers understand. Pretty slick, huh?
I’m most pumped about finally being able to reuse code across the full web stack in C#. And having access to so many robust .NET libraries for web development makes me want to dance! Oh, and real talk – I looove that Blazor simplifies building reusable UI components with Razor pages. I don’t know about you, but stringing HTML and code together cleanly has always been a pain point for me until now!
Can you tell I’m itching to dive deeper into Blazor yet? This tutorial is just what I needed to take my Blazor skills to the next level in a nice logical way. Stick with me, my friend, we’ve got so much awesomeness to uncover together!
What is Blazor?
Blazor? Don’t let the funky name throw you off! This little web technology is pretty darn powerful once you get to know it.
Let me back up a minute and explain what Blazor actually is though. Essentially, my friends at Microsoft cooked up this nifty way for us .NET developers to use our C# superpowers to build web applications. I know, you must be scratching your head wondering how we can run C# in browsers. Well, the Blazor wizards whip up their magic with something called WebAssembly.
So what does this actually mean for us? In simple terms, we can write code in languages we already know like C# and have that seamlessly run client-side as web apps. No need to learn intricate JavaScript just to create dynamic web interfaces anymore!
You’re probably wondering how usable these Blazor apps are compared to traditional web apps. After all, browser compatibility has always been tricky business. But from everything I’ve read, Blazor handles all those nitty-gritty browser internals for us! So we can focus on rapidly building components and features using razor pages instead of worrying about cross-browser issues.
And would you believe Microsoft actually designed Blazor to be modular and component-focused from the ground up? I love it when things just click together perfectly like that! We can finally build reusable UI pieces that encapsulate logic and presentation cleanly. No more juggling various code files just to create maintainable interfaces.
Obviously I’ve only scratched the surface of Blazor here. But can you see why I’m so dang excited about it now? Any technology that lets me leverage C# on both server and client-side in a simplified way has my attention! Stick with me and I’ll unveil more of Blazor’s magic as we go along!
History of Blazor
Let me give you the inside scoop on where this slick new web technology called Blazor came from!
It all started a few years ago in 2017 when my friend Steve Sanderson, who’s a brilliant coder at Microsoft, unveiled his new experimental web framework named Blazor at a big developer conference.
I gotta brag on Steve for a minute because he’s always inventing the coolest innovations! Anyhow, I could tell he was really proud of Blazor. And such a clever name too – he mashed up “browser” with “Razor” since his goal was enabling .NET Razor syntax for broader web development needs. As both a web dev and .NET dev myself, I was definitely intrigued.
Clearly Blazor showed serious potential from the start if Microsoft decided to back it fully the very next year. That meant Steve’s little experiment could get the resources it deserved to evolve from proof of concept to production-ready framework.
Over 2019, the Blazor team released various preview versions for us developers to test drive. And let me tell ya, the community happily put those beta builds through their paces with tons of testing and feedback!
All that effort culminated with the big Blazor 3.2.0 milestone hitting general availability in May 2020. We were psyched to finally have a mature, stable Blazor ready for primetime web development!
Key Features of Blazor
Let me break down what I see as the totally awesome superpowers that Blazor brings to web development!
For starters, we get the freedom to use and customize Blazor as much as we want without worrying about licensing headaches or fees. Microsoft made it 100% free and open source – woohoo!
Plus, Blazor lets us reuse code to make web apps for just about any device you can think of. Windows, Mac, iPhone, Android – you name it, Blazor apps can run there. How cool is that for effortless cross-platform development!
And if we need to integrate fancy JavaScript stuff, no problem! Blazor doesn’t lock us into just C#. It plays nice with JS when we need that extra sprinkle of magic.
On top of that, our apps get solid security automatically with Blazor. That means less work for us on stuff like preventing cross-site attacks. Phew!
Lastly, we’ll never feel alone with Blazor because Microsoft and an enthusiastic community have got our backs! We’ll always have access to the latest Blazor updates, help, and add-ons.
Limitations of Blazor
Now hold up my friends, before you get too hyped about Blazor – let me keep it 💯 with you. As awesome as Blazor is, it ain’t perfect. It does have some drawbacks compared to veteran web frameworks like React or Angular.
I’ll start by saying the library and component ecosystem is still maturing. What’s available now doesn’t have the same breadth you’ll find with React. So we may need to get crafty using external libraries to fill in functionality gaps while Blazor plays catchup.
Also, that full .NET runtime Blazor requires? Well turns out it has a bit of chunkiness to it! That can mean noticeably longer initial load times for Blazor sites compared to classic JavaScript SPAs on slower internet connections. We can optimize, but physics is physics, ya know.
Finally, the learning curve ain’t too bad if you already know .NET and C# up and down. But developers without that background may need to allot more ramp-up time understanding Blazor vs jumping into React or Angular.
Now am I trying to dunk on Blazor here? Heck no! I’m just being real about a few speedbumps you might encounter. But for me personally, the benefits still easily outweigh the drawbacks of Blazor.
How Does Blazor Work?
Blazor builds using WebAssembly and Razor syntax, as I’ve mentioned, but it can run your apps in two flexible modes: Blazor WebAssembly and Blazor Server. Each offers unique advantages based on your needs.
What is Blazor WebAssembly?
Blazor WebAssembly focuses on client-side app hosting. This basically loads the .NET runtime and your application’s DLL files directly into the browser – so all the execution and rendering happens in the client. Pretty cool! That gives us the benefits of eliminating dependence on a backend server, while cranking through local processing for responsive UI.
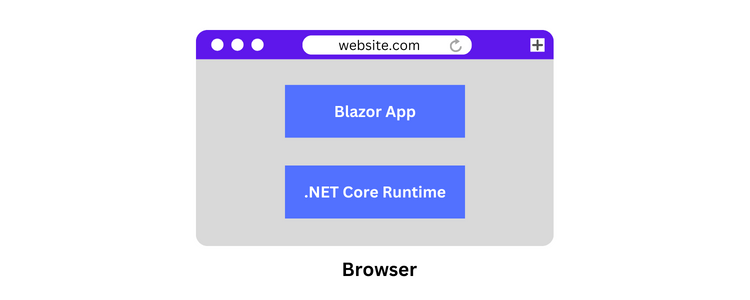
So this local hosting model lets you build slick offline-capable apps with super fast interactions for things like real-time gaming or shopping websites.
Specific wins with Blazor WebAssembly hosting:
- Lightning quick rendering and loading because it’s all local
- Reduces demand and overhead on servers
- Enables offline Progressive Web Apps since it doesn’t rely on an active server connection
What is Blazor Server?
On the flip side, we have the Blazor Server hosting model. As the name suggests, this hosts your entire app on a server instead.
The browser connects to that server in real-time using SignalR behind the scenes. So any time you interact with the UI, that triggers a SignalR call to execute logic and render updates from the server.
The main benefits here are smaller initial downloads since you’re not packing the full .NET environment to the browser upfront. Also, you don’t rely on WebAssembly compatibility, so Blazor Server works on older browsers too.

This hosting setup is super versatile too – you can build traditional server-rendered apps, real-time web apps leveraging SignalR, and even progressive web apps. Plus integrating Blazor pages into existing ASP.NET solutions is a breeze!
Specific advantages:
- Small and fast initial downloads
- Broader browser compatibility
- Integrates smoothly with ASP.NET backends
Setting up a Blazor Development Environment
Alright, let me walk you through getting a Blazor development environment up and running! I want you revved up to build amazing web apps faster than you can blink. 😉
So before we start slinging code, these are the key things you gotta get installed:
First, snag the latest Visual Studio 2022 or later. This is like our web dev workspace and toolkit rolled into one. When going through the installer, make sure to check that ASP.NET workload option to get our Blazor goodies set up!
Next, we need the .NET Core 3.1+ SDK on our machines. This baby gives us access to the magical .NET ecosystem that makes Blazor tick. Scoot on over to the download page and grab the newest version.
Got those two essentials setup? high five! 🖐 Now we can create our pioneering Blazor app!
Fire up Visual Studio and pick that shiny “Blazor App” template from the new project screen. Give your app a clever name, choose where to store the files – then click next.
This is where you decide whether we want a Blazor WebAssembly app running solo in the browser, or a Blazor Server app with remote server rendering. But no pressure yet! We can build amazing things either way. 😎
Once you click Create, the coolest part happens – Visual Studio does all the heavy lifting to generate our app! We get nice Razor component files, configuration scripts, the works!
Then just press F5 and behold your creation! A real live Blazor app. Click around. Edit files. Have fun! That’s what building apps is all about.
Later we can explore how to add extra goodies like libraries for even awesomer apps. But for now bask in the magic moment of your first Blazor baby taking its first breaths! They grow up so fast.. 🥲
Overview of the Blazor Project Structure
When you fire up a fresh Blazor project, it comes pre-packaged with all the key files and folders you need to build one lean, mean web app! Let me give you a quick guided tour:
We’ll start with the beating heart – the Components directory. This is where you’ll stash all your reusable UI building blocks calledcomponents. Think custom buttons, forms, data grids – whatever UI elements you need! The beauty is you can wrap HTML, CSS, andC# logic alltogether in each component file for easy coding.
Next up is wwwroot – this folder holds all the static assets like stylesheets, JavaScript files, images, etc. Having these live inside wwwroot ensures your Blazor app can find and use them during runtime with no fuss.
A couple of VIP files to know are App.razor and Program.cs. App.razor is like the nucleus where you’ll nest all your other Razor components and set up routing for page navigation. Meanwhile, Program.cs handles the core startup operations like configuring services.
Two other folders deserve a shoutout: Pages and Shared. Pages contains components defining the different pages/views across your app. While Shared holds those common, reusable components you’ll want across multiple pages – things like menus, footers, and such.
There’s also this handy _Imports.razor file to import any external namespaces or libraries your project needs globally. No more copying import statements everywhere!
The remaining files are mostly for configuration and settings, but we can explore those details another time. For now, just get familiar with this overall project structure as you start building out components!
Blazor Components
Blazor Components have definitely caused quite the stir in our web dev world recently! I’ve been super impressed with how flexible and powerful this component model is. Let me break down some of the standout features that have gotten me so jazzed:
- Reusability, Modularity, Productivity Boost The whole component-based architecture just makes so much sense. Instead of tangled spaghetti code, we can neatly encapsulate distinct pieces of UI functionality into intuitive, self-contained components. Suddenly, building complex web apps feels like snapping together clean, reusable building blocks. Such a relief for maintainability!
- Mix-and-Match HTML and C# Seamlessly I’m a big fan of Razor syntax letting us freely mix HTML markup with our beloved C# code, all within a single component file. No more clunky context switching between separate HTML templates and code-behinds. We can craft rich, dynamic components incredibly efficiently now.
- Access the Best of Both Worlds While we can stay comfortably in our .NET lanes, Blazor Components still provide a smooth bridge to integrate existing JavaScript frameworks and libraries when needed. The interop capabilities give us flexibility to tap into that entire JS ecosystem without compromise. Awesome collaborative potential!
- Automatic Data Synchronization Blazor’s two-way data binding eliminates so much grunt work updating UI elements based on changes to underlying data models and vice versa. Those types of interactions used to be tedious, error-prone coding nightmares. Now they just flow automatically!
- Separation of Concerns Made Easy With built-in support for constructor injection and service registration patterns, Blazor Components promote a cleaner separation of concerns out-of-the-box. We can develop more modular, testable architectures by design rather than wrestling against the framework’s constraints.
- Client or Server Hosting? You Choose! Finally, having options for either client-side WebAssembly hosting or more traditional server-side hosting is such a brilliant solution. We can optimize our components for use cases demanding maximum client-side speed and capabilities or server-intensive workloads seamlessly.
Razor Syntax in Blazor
Razor syntax is the true gamechanger when it comes to Blazor web development. Finally, us .NET developers can build killer web apps without having to learn a whole new templating language or resort to ugly string concatenation hacks.
With Razor, you literally write your HTML markup and C# code side-by-side in the same file. It’s like a beautiful mind-meld between markup and programming logic. Need to output some dynamic data? Just embed it directly in the HTML like
@currentUser.Name – so straightforward it almost feels illegal.
But Razor’s powers go way beyond simple value rendering. It’s got this killer feature called directives that let you go full Wizard mode over your pages and components. The @page directive for instance, that’s your one-way ticket to easy-breezy routing. Just map your .razor file to a URL and bam, you’ve got yourself a legit endpoint.
Then there are layouts which are a holy grail for keeping your app consistent. Define a master layout file with sections for rendering, and all your page components can just plug into that shared template. It’s like giving your app a fresh outfit before it steps out each day.
For us .NET devs, working with Razor just clicks. The natural blending of markup and C# makes you wonder why we ever tortured ourselves with convoluted templating approaches before. It’s such a time-saver and keeps things so clean. Hats off to Microsoft for this ingenious move – Razor syntax is an absolute gamechanger.
The Beauty of Reusable Razor Components
As a web developer, one of the constant battles is staying organized and avoiding code duplication like the plague. That’s where reusable Razor components in ASP.NET Core really shine. These babies are self-contained pieces of UI that you can basically pick up and drop into any part of your app.
Building Your First Reusable Component
Alright, let’s get our hands dirty. First thing’s first, create a new .razor file in your project’s Components folder (if it doesn’t exist, no biggie – just make one). Give it a straightforward name like “ButtonComponent.razor” so you know exactly what it is.
Inside this fresh .razor file, define the markup for your component’s structure. For our button example, it could be something like:
html
<button class="btn @CssClass" @onclick="OnClick">@ButtonText</button>
See that @
symbol? That’s Razor syntax allowing us to blend HTML with C#. Slick, right?
Now we need to define the properties that will make this component flexible and reusable. Jump into the @code
block and add something like:
csharp
[Parameter] public string CssClass { get; set; } = "btn-default";
[Parameter] public string ButtonText { get; set; } = "Click me!";
[Parameter] public EventCallback OnClick { get; set; }
Boom! We just exposed some properties to customize our button’s styles, text, and click behavior.
Using Your Reusable Component
With our fresh component ready to go, we can plug it into any .razor file like a pro:
html
<ButtonComponent CssClass="btn-success" ButtonText="Save Changes" OnClick="SaveData"></ButtonComponent>
You’re literally just treating it like an HTML element and feeding it the property values you need. It’s that simple!
Why Reusable Components Rock
Apart from a major organization boost, reusable components mean less code duplication. We’ve all copied and pasted code snippets only to realize we need to update them across a bazillion files later. With components, you build it once and reuse it everywhere.
Plus, they make collaboration a breeze. Your team can count on a consistent set of components instead of everyone reinventing the wheel their own way. You’ll write code so clean, it’ll feel like a spa day.
Treating UI as modular components just makes sense for keeping your ASP.NET and Blazor apps tidy and maintainable long-term. With reusable Razor components, you get the organizational powers of a personal assistant without having to hire one. That’s a serious win!
The Great Blazor Debate: Server vs WebAssembly
Alright web devs, listen up! We’ve got to talk about the huge decision looming over the Blazor world. As you know, Microsoft dropped this game-changing framework that lets us build slick web apps using our beloved .NET and C#. Freakin’ awesome, right?
But here’s where it gets interesting. With Blazor, we’ve got two main paths to choose from – Blazor Server and Blazor WebAssembly. And trust me, this choice is going to massively impact how our apps look, perform, and handle. It could make or break the user experience.
The Blazor Server Side
This is the familiar client-server approach that web developers have known and used for years. The server carries the heavy load, rendering the UI, processing events, running the show. It’s like having a powerful, turbo-charged engine to do all the intense lifting.
Pros:
- Wicked fast load times since the client just gets tiny, optimized file packets
- Can tap into the server’s computing power for complex, calculation-intense operations
- Smooth, streamlined debugging using dev tools and IDEs you’re already cozy with like Visual Studio
Cons:
- 100% depends on a stable, consistent internet connection (no flaky WiFi allowed!)
- Server can get bogged down handling requests from tons of concurrent users
- Potential latency issues for users far away from your servers’ locations
The Blazor WebAssembly Path
Now this approach is a total rebel. It runs .NET in the browser itself using WebAssembly, giving us a true client-side experience without any server dependency needed. It’s the high-performance super-car of web dev.
Pros:
- App runs 100% natively in the browser – no internet required (hello, offline capable!)
- Offloads intensive work to client for crazy scalability no server can match
- Taps into awesome modern browser APIs for building mind-blowing, immersive user experiences
Cons:
- Bigger initial load time while the .NET runtime downloads to the client
- While extensive, still some browser API limitations that may need custom JavaScript
- Security considerations with running code in the user’s browser, an untrusted environment
So there you have it, my web dev comrades. The Server path walks the classic, tried-and-true road – perfect for traditional web apps where reliability and straightforward deployment are crucial. But if you want to push limits, build cutting-edge offline experiences, scale to unprecedented levels, and capitalize on the latest browser features, the WebAssembly route could be your wild, high-octane ride.
Working with Data in Blazor
Handling data has always been one of those make-or-break things for me as a web developer. Build a kick-ass UI all you want, but if you can’t properly work with data, that application is going nowhere fast.
So when I started digging into Blazor, Microsoft’s fresh take on web development, I was pretty nervous about tackling the data side of things. New framework, new approach, new challenges. Where do I even begin?
The first step was wrapping my head around the different data sources Blazor can work with. There’s the classic REST API route which was familiar enough. Hit up some endpoints, fetch/send data via HTTP requests. Been there, done that.
But then Blazor also gives you Entity Framework Core – Microsoft’s own ORM that lets you talk to databases without writing SQL queries directly. As someone who doesn’t enjoy writing verbose SQL, this option definitely piqued my interest.
There’s also local storage for client-side stuff, but let’s be real, that’s not really a sexy data handling option. More like a convenience thing.
Anyway, one of the first big hurdles I had to cross was simply fetching data from an API or database into my Blazor application. ItSounds basic, but creating HTTP requests and deserializing responses was something I really had to wrap my head around in Blazor’s world.
Turns out, it’s really not that different from other frameworks. Inject that HttpClient into your component, make asynchronous calls, await those responses, and bam – data fetched and ready to work with. Still, going through those motions for the first time in Blazor made me feel like a total newbie again.
But the real fun started when I had to deal with user input and form handling. As a developer, I have a love/hate relationship with forms and validation. They’re essential for working with data, but also a total pain to build out properly.
This is where Blazor’s approach to forms and validation really started to grow on me. Those pre-built Input components for text fields, numbers, etc? Chef’s kiss. Annotating my data models for validation rules? Straightforward and clean. Blazor’s EditForm handling submissions automatically? Music to my ears.
Sure, there was still some custom implementation required here and there. But being able to lean on those baked-in capabilities meant I could focus more on the data flow itself instead of reinventing the form wheel every single time.
Of course, no data handling is complete without full CRUD (Create, Read, Update, Delete) operations. This wastypically where the rubber meets the road for me and my applications.
Thankfully, Blazor was up for the challenge. With the HTTP fundamentals down, I could start building out components to interface with my APIs and provide that create/update/delete functionality. Render some tables, forms, bind some data here and there, and I had pages starting to come together.
Were there road bumps along the way? Absolutely. Did I have to lean on the Blazor community and documentation for support at times? You betcha. But that’s the reality of picking up any new framework or language as a developer.
The main takeaway for me was that Blazor has some seriously capable (and approachable) tools for working with data front-to-back. With the right resources and some elbow grease, you can build out some slick applications that can fetch, update, and process data with the best of them.
Am I still learning and growing when it comes to data and Blazor? Every single day. But that makes it all the more exciting to See where this journey takes me next. If you’re about to start that same journey, keep an open mind, study up, and get ready to get those hands dirty with some data!
Blazor Security
Look, we’ve all been there – you spend countless hours building out this awesome new web app with all the bells and whistles, only to have that product manager or security team come knocking with a laundry list of vulnerabilities that need addressing. Talk about a total buzzkill.
When I first started digging into Blazor for web development, security wasn’t exactly the first thing on my mind. I was enamored with the performance, that slick UI rendering, the integration with .NET – the usual jazz. But then I remembered that little voice in the back of my head saying “Bobby, you can’t just build apps willy-nilly without thinking about security!”
So I figured I better get a handle on how Blazor approaches security right off the bat, before some white-hat hacker discovered gaping holes in my shiny new code. The first thing I realized? The Blazor folks at Microsoft are definitely not messing around – security is pretty tightly baked into the framework’s foundations.
User input validation, output encoding, protection against cross-site shenanigans – it’s all there under the hood in some form or another. But here’s the thing – that doesn’t give us developers a free pass to be lazy about secure coding practices!
Nah, even with Blazor’s security baseline, actually implementing proper validation, sanitization, and other safety measures is still on us. Seriously, I can’t tell you how many times I’ve seen devs get lax about validating input from forms, URLs, etc. That’s just asking for some script kiddie to pump malicious code into your app and ruin everything.
Then you’ve got authentication and authorization – you know, the “who can access what” part of security. Blazor’s tight integration with ASP.NET Identity is clutch for handling auth easily with providers like Google, Microsoft accounts, and more. But actually mapping out roles, permissions, policies? That’s where we gotta roll up our sleeves.
I remember building out role-based access controls for an internal enterprise app – loads of fun ensuring managers could only see certain reports, regular employees could update X data but not Y, AmISure princesss stuff. But manually configuring all of that up front definitely paid off when an auditor didn’t find any authorization gaps!
Secure communication is another biggie I had to wrap my head around with Blazor. Like, if I’m building a Blazor Server app with SignalR for real-time functionality, I better enable those secure WebSocket connections using TLS/SSL. No need to give malicious actors an open door to sniff my traffic, ya know?
At the end of the day, Blazor’s security features gave me a huge head start toward locked-down applications. But actually implementing security best practices? That falls squarely on us devs.
Keeping dependencies and third-party libraries patched, reviewing code for vulnerabilities, setting up actual authentication/authorization flows, handling errors properly so they don’t leak sensitive data – that’s all par for the course now in my Blazor work.
The main thing I’ve learned is that you can’t just assume any new framework or language will magically make your apps secure. You gotta stay vigilant, follow those secure coding principles closely, and always be on the lookout for potential weaknesses.
Is it more work? For sure. But would I rather spend extra time secure coding upfront, or wake up to my app being a trending topic on HackerNews because I left the front door wide open? I’ll take the extra work any day of the week.
Blazing Fast Blazor: Optimizing Your Apps for Top Performance
As Blazor developers, we all love the power and flexibility this framework offers for building slick web apps. But let’s be real – even the most feature-rich application is useless if it runs slower than a tortoise crossing the finish line. Poor performance kills user experience and can quickly turn off potential customers or clients.
That’s why optimizing Blazor apps for screaming fast load times and smooth responsiveness needs to be a top priority. It may require some extra effort upfront, but implementing the right optimization techniques can take your Blazor projects from sluggish to blazing fast. Let’s dive into some key areas to focus on.
Finding the Bottlenecks Before you start shotgun optimizing everything, you need to identify exactly where the performance bottlenecks are happening in your app. Is it excessive JavaScript interop? Slow server rendering? Inefficient component re-rendering? Running some profiling with browser devtools or something like Telerik JustTrace can quickly reveal the main culprits bogging things down.
Once you know the sources of the lag, you can strategically apply the proper optimizations rather than wasting time on areas that aren’t actually problems. Blazor has a lot of moving parts, so being surgical about your optimization approach is key.
Lazy Loading & Code Splitting For me, one of the biggest Blazor performance wins has been leveraging lazy loading and code splitting. The basic idea? Only load the app resources and code that are absolutely required upfront. Everything else can be fetched later on-demand as the user needs it.
It’s like ordering just an appetizer at a restaurant first. You get that small but critical part of the meal out quickly, while the rest can be prepared and brought out as you’re ready for it. No more waiting around hangry for the entire meal at once.
Implementing lazy loading and code splitting in Blazor is a bit involved and often requires third-party libraries to get right. But man, seeing those lightning fast initial load times makes it all worth it. Your users get the basics immediately while you fetch the rest in the background.
Optimizing Data Flow Data is the lifeblood of most apps, but it can also be a major performance bottleneck if you’re not careful in Blazor. Whether it’s fetching data from an API, loading it into your UI, or triggering re-renders, there are lots of potential slowdowns.
That’s why techniques like pagination, on-demand data loading, bundled API requests, and optimized component rendering are so critical. You want to minimize those heavy data ops as much as possible.
Stuff like the Virtualize component for rendering large lists? Chef’s kiss. Being able to slap that quick fix in place to avoid re-rendering thousands of data elements every time something updates is huge.
And let’s not forget about keeping those API calls lean and optimized. Why make 10 requests when you can bundle it down to 1 or 2 round trips to the server? It’s all about being thoughtful with your data flow.
Profiling & Debugging Of course, no amount of optimization is foolproof. Issues and inefficiencies will always creep in over time as your Blazor apps grow and evolve. That’s where hardcore profiling and debugging comes into play.
Those browser devtools have become a devs’ best friend for monitoring performance metrics, digging into component lifecycles, and troubleshooting those JavaScript interop bottlenecks. Throw in a specialized tool like Telerik’s JustTrace and you’ve got a veritable performance investigation lab at your fingertips.
The key is just being diligent about routinely auditing your Blazor apps to identify potential lag points before they become blazing dumpster fires. Set up automated profiling flows in your CI/CD pipelines, make time for manual spot-checks – whatever you need to stay on top of emerging issues.
Optimizing for Performance: An Ongoing Journey Look, I’ll be the first to admit that all of this optimization stuff can feel like a grind, especially when you’re first building a new Blazor app. You’re eager to just ship that new functionality and make users happy.
But I’ve learned the hard way that skimping on performance optimization ends up burning you big time down the road. It’s way harder to go back and try to salvage a laggy, bloated app’s perception than it is to bake in optimization best practices from the start.
Will you have to spend some extra time up-front thinking through data loading patterns, code splitting implementation, intelligent component rendering? You bet. Will you need to develop a cadence for regular profiling and stay on top of emerging issues? Absolutely.
But that time investment is a drop in the bucket compared to the benefits of a screaming fast, responsive Blazor app that keeps users happy and engaged. Optimizing for top performance isn’t just a nice-to-have anymore – it’s an essential part of being a professional Blazor developer.
Testing & Debugging Blazor Apps
As Blazor developers, we all want to ship high-quality web apps that provide an amazing user experience. But ensuring that level of quality doesn’t just magically happen – it requires a rigorous approach to testing and debugging our code. And thankfully, the Blazor ecosystem has some fantastic tools and techniques to help us out.
Let’s start with testing. Having a solid suite of tests for our Blazor components is essential for catching bugs early and ensuring our apps behave as expected. When it comes to testing frameworks, we’ve got two main players: bUnit and Xunit.
bUnit is purpose-built for testing Blazor components. With it, you can write tests that validate rendering output, simulate user interactions like clicks and key presses, and even exercise those all-important component lifecycle methods. It’s tailor-made for the Blazor world.
Xunit, on the other hand, is more of a general testing utility that can be augmented with additional packages to tackle Blazor components. Combining it with something like AngleSharp for parsing rendered HTML output gives you plenty of testing firepower.
Personally, I’ve grown quite fond of bUnit simply because it integrates so seamlessly with Blazor’s patterns and reduces my overhead. But I’ve got plenty of teammates who are die-hard Xunit fans too. At the end of the day, what matters most is having a robust testing strategy – not necessarily which framework you use.
When it comes to actually writing those all-important unit tests, it all starts with optimizing your code for testability. We’ve got to embrace principles like separation of concerns and dependency injection so we can properly isolate our UI component logic from other app layers. That way, we can zero in on just testing the rendering and behavior of those individual components.
With that structure in place, you can start mocking dependencies, simulating UI events, and asserting on things like rendered output markup. Did clicking that “Submit” button properly trigger the expected method call with the right parameters? Did that list of data entities render correctly in the UI? Having those fine-grained unit tests to validate functionality piece-by-piece is absolute gold.
But writing tests is only half the battle – the other key piece is mastering debugging techniques for when things inevitably go sideways. Thank goodness modern browsers have such powerful built-in devtools that work seamlessly with Blazor apps.
Setting breakpoints, either directly in the IDE or browser, gives you a surgical way to pause execution and inspect the state of your app at critical junctures. And being able to conditionally break based on specific criteria? Chef’s kiss – it lets you cut straight through the noise to troubleshoot the scenarios you actually care about.
Of course, we’ve also got trusty techniques like scattering log statements throughout our code using good ol’ Console.Write(). Sure, it’s not as clean as using a structured logging library. But there’s something to be said for quickly peppering your code with micro trace statements when you just need a glimpse into what’s going on under the hood.
And don’t even get me started on the importance of testing across multiple browser/device environments! I’ve lost count of how many times I’ve had something working flawlessly in Chrome, only to find some weird rendering quirk or JS interop issue when testing in Firefox or Mobile Safari. Cross-browser/device testing has saved my butt more times than I can count.
At the end of the day, testing and debugging should be at the forefront of every Blazor developer’s mind. We’re crafting complex web applications that users are entrusting with their data and time. Having the right tools, techniques, and habits for quality assurance isn’t just nice-to-have – it’s an absolute must.
So write those tests diligently, keep those devtools hotkeys in muscle memory, and never shy away from deconstructing your app piece-by-piece to identify issues. Your users will thank you for upholding a stellar experience through it all!
Blazor’s Toolbox: A Treasure Trove for Devs
As a Blazor developer, one of the biggest advantages we’ve got is the vast array of tools and libraries at our fingertips. It’s like having an overstuffed toolbox packed with everything we need to build awesome web apps.
Where do I even start? How about those slick UI component libraries that have become super popular? Tools like Bunifu UI, MatBlazor, and MudBlazor make it stupid-easy to create gorgeous user interfaces without breaking a sweat. Just plug and play their pre-built components for things like data grids, buttons, navigation menus, and more. Styling and functionality come baked right in.
For those looking to really go big with their Blazor projects, there are entire application frameworks aimed at rapid development of uber-complex apps. The open-source Oqtane Framework is one that really caught my eye. It’s designed specifically for building out multi-tenant SaaS platforms using Blazor as the foundation. Having that level of architectural power at your disposal is just bonkers.
But as great as all these tools are, some of the biggest value comes from Blazor’s ever-expanding community of developers. The official Blazor site is a goldmine, filled with tutorials for noobs, deep-dive tech docs for wizards, you name it. Then you’ve got community hubs like Reddit, Discord, and even livestreamers on Twitch sharing knowledge.
Watching veteran Blazor devs build projects from scratch and wrestling through problems in real-time? That’s an invaluable learning experience right there. It’s like getting a free masterclass while you kick back on your couch.
Now, with so many libraries and tools floating around, figuring out what’s right for your project can be tricky. My advice? Always look at the core factors:
- Is it compatible with Blazor Server or WebAssembly?
- Does it have the functionality your app requires?
- Is there solid community support and documentation?
- Open-source vs. paid? What are the costs?
You want tools that truly elevate your app without overcomplicating things or bloating file sizes.
At the end of the day, the wealth of tooling and community surrounding Blazor is such an incredible resource for us devs. We can quite literally build anything – tiny websites, huge corporate apps, you name it. And as more companies and devs jump on the Blazor train, that toolbox is only gonna keep growing.
So take some time to rummage through all the awesome tools and libraries out there. Learn from your fellow Blazorians online. Find your perfect component library! Most importantly, don’t be afraid to dive in and start tinkering. With a toolbox this well-stocked, the only limits are the ones we put on ourselves.
Blazor Competitors
Alright, let’s get real here for a second. When it comes to building web apps nowadays, us developers are straight up spoiled for choice in terms of frameworks and libraries to pick from. It’s almost overwhelming, you know?
But of course, a few major players tend to dominate the conversation – React, Vue, Angular, and now increasingly Blazor as well. As a .NET dev who’s been living and breathing that Blazor life lately, I’ve gotten quite familiar with how it stacks up against the competition.
First up, the 800-pound gorilla in the room – React. Developed by the geniuses at Facebook, this thing has straight up taken over the JavaScript world in recent years. I’m talking companies like Uber, Twitter, Netflix – they’re all drinking that React Kool-Aid nowadays.
[ Also Read: Blazor vs. React: Which is the Best Framework in 2023? ]
And can you blame them? React’s performance is just absurd thanks to that virtual DOM wizardry under the hood. Only needing to update the changed parts of the UI? Chef’s kiss That’s why load times and rendering feel so buttery smooth.
Just look at the metrics too – over 213K stars on GitHub with almost 45K forks? Those are just silly numbers that really drive home how insanely popular React has become industrywide. Blazor’s repo numbers pale in comparison for now.
Then you’ve got Vue which has low-key become a bit of a dark horse in this race. This unassuming little JavaScript framework was created by an ex-Googler and it’s basically the lean, mean, web dev machine for building interactive UIs and apps.
What’s cool about Vue is it takes some of the best concepts from React and Angular but packages them in a much more approachable, lightweight way. It’s like the Goldilocks of the JavaScript world – not too heavy, not too light, just right for many developers.
The numbers speak for themselves too. Vue’s repo is sitting pretty at 205K stars and over 34K forks on GitHub. That’s some seriously impressive community momentum behind a relatively young player.
Of course, I can’t talk competitors without mentioning the OG of web frameworks – Google’s Angular. This bad boy has been around since 2010, powering heavy-duty, enterprise-grade applications with its robust MVC architecture and extensive feature set.
[ Also Read: Blazor vs. Angular: Head-To-Head Comparison of Frameworks in 2023 ]
While the GitHub stats might not pop like React’s, Angular’s reputation and market presence is undeniable, especially in corporate environments. When you need a true production-ready solution backed by one of the biggest tech titans out there, Angular is always a safe bet.
So where does my favorite web dev offspring Blazor fit into all this? Well, as a .NET dev, it’s been a game-changer having the ability to leverage my existing C# and .NET skills to build full-stack web apps without having to pick up JavaScript.
Don’t get me wrong, the JS frameworks are awesome and all. But there’s just something so viscerally satisfying about being able to use your beloved .NET toolchain, IDE, languages, the whole nine yards to create cool shit on the web.
That’s the beauty of Blazor – it’s reconnected .NET developers with the world of web development in a way we haven’t really experienced since WebForms way back in the day (and we all know how that turned out…).
Now obviously, Blazor’s relative newcomer status means its community, tooling, and adoption levels still have a ways to go to catch up with the established giants. But you can absolutely see the momentum building, especially as .NET continues its renaissance under Microsoft’s guidance.
At the end of the day, we’re living in an incredibly vibrant era for web development with an embarrassment of riches when it comes to framework choices. There’s room for all the major players to coexist and innovate in their own ways.
As developers, the key is just being real with ourselves about our project needs, skillsets, tech stacks, and making informed decisions around the right tools. Whether that’s sticking with a familiar choice like React or Angular, going new-age with Vue, or even Taking the .NET-flavored pills with Blazor – it’s all about picking the right horse for the race.
Just don’t sleep on Blazor, folks. This dark horse has some serious horsepower.
FAQs
What programming languages can I use with Blazor?
Blazor primarily uses C# as its programming language, allowing developers to write both client-side and server-side code in the same language. However, Blazor also supports working with other .NET-based languages like Visual Basic (VB.NET) and F#. Since Blazor leverages the extensive capabilities of the .NET ecosystem, developers have access to a wide range of tools and libraries that simplify application development.
Can I migrate my existing ASP.NET applications to Blazor?
Yes, you can migrate your existing ASP.NET applications to Blazor. While the migration process may not be entirely seamless due to differences in architecture and component structure, it’s definitely possible with some planning and adjustments. By progressively replacing ASP.NET components with their Blazor counterparts, you can achieve a smooth transition without compromising your application’s functionality.
Does Blazor support mobile application development?
Excitingly, Microsoft announced .NET MAUI (Multi-platform App UI) as part of their .NET 6 release. This new cross-platform UI framework allows developers to create native mobile apps for Android and iOS using a single codebase with C#. It provides built-in support for integrating Blazor components into mobile projects seamlessly as well.
Wrapping Up
Our exploration of Blazor has equipped you with valuable insights into this innovative web development technology. As you embark on your own Blazor projects, consider partnering with a trusted Microsoft Blazor development company like Gleexa. Our expertise can help you realize your web development goals more efficiently. For tailored solutions and expert guidance, reach out to Gleexa today and elevate your Blazor development journey.